How to set content disposition headers for express nodejs apps
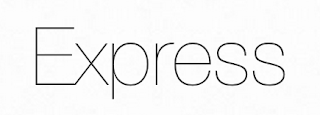
In express nodejs apps you can force the user agent "browser" to rather download content instead of displaying or attempting to render given content within the browser. In this example assuming you're using express.static to serve content pass an Object as second argument to express.static function An Object with configuration parameters; const config = { setHeaders: res => res.set('Content-disposition', 'attachment') } In your code replace "path/to/media/" with the path pointing to the static content. app.use('/media/', express.static('path/to/media/', config)) To test try accessing content at /media/*