How to get first and last character of a string using 'String.startsWith' and 'String.endsWith' in JavaScript
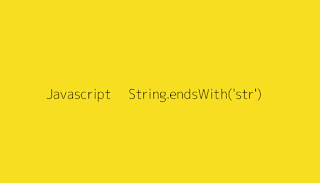
String starts with is an emcscript es6 feature that allows JavaScript developers to test a string if the first character of a string matches the given criteria , Take for instance you have a string, you want to test if it starts with characters '#' const str = "#JavaScript" cons strr = "I love #kotlin" str.startWith('#') // true strr.endsWith('#kotlin') // true strr.startWith('#') // false str.endsWith('#kotlin') // false Both functions return a boolean true for a match and false if there's no match. endsWith does what startsWith does except that it does it at the end of the string